pterm
pterm: Pretty Terminal Printer
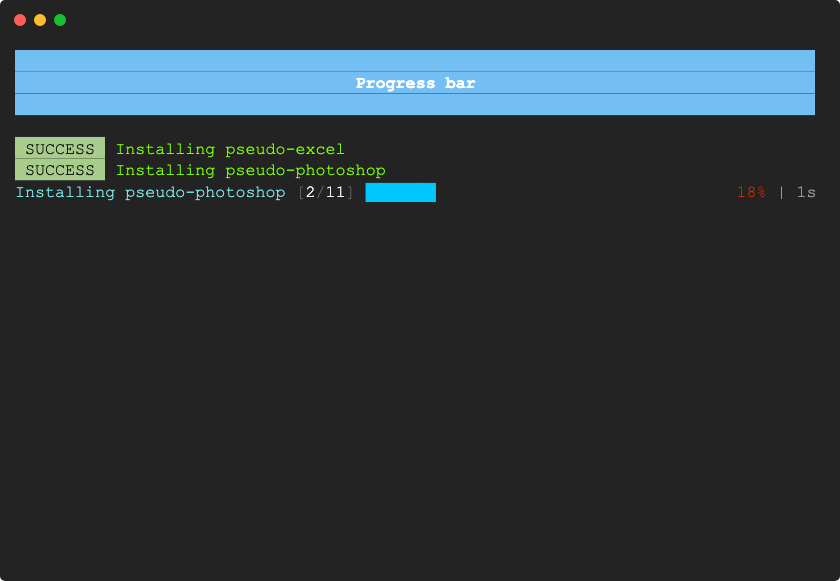
⭐ Main Features
Feature | Description |
---|---|
🪀 Easy to use | Our first priority is to keep PTerm as easy to use as possible. With many examples for each individual component, getting started with PTerm is extremely easy. All components are similar in design and implement interfaces to simplify mixing individual components together. |
🤹♀️ Cross-Platform | We take special precautions to ensure that PTerm works on as many operating systems and terminals as possible. Whether it’s Windows CMD , macOS iTerm2 or in the backend (for example inside a GitHub Action or other CI systems), PTerm guarantees beautiful output! |
🧪 Well tested | PTerm has a 100% test coverage, which means that every line of code inside PTerm gets tested automatically We test PTerm continuously. However, since a human cannot test everything all the time, we have our own test system with which we currently run 28774 automated tests to ensure that PTerm has no bugs. |
✨ Consistent Colors | PTerm uses the ANSI color scheme which is widely used by terminals to ensure consistent colors in different terminal themes. If that’s not enough, PTerm can be used to access the full RGB color scheme (16 million colors) in terminals that support TrueColor . |
📚 Component system | PTerm consists of many components, called Printers , which can be used individually or together to generate pretty console output. |
🛠 Configurable | PTerm can be used by without any configuration. However, you can easily configure each component with little code, so everyone has the freedom to design their own terminal output. |
✏ Documentation | To view the official documentation of the latest release, you can go to the automatically generated page of pkg.go.dev This documentation is very technical and includes every method that can be used in PTerm. For an easy start we recommend that you take a look at the examples section. Here you can see pretty much every feature of PTerm with example code. The animations of the examples are automatically updated as soon as something changes in PTerm. |
Printers (Components)
Feature | Examples | – | Feature | Examples |
---|---|---|---|---|
Bar Charts | Examples | – | RGB | Examples |
BigText | Examples | – | Sections | Examples |
Box | Examples | – | Spinners | Examples |
Bullet Lists | Examples | – | Trees | Examples |
Centered | Examples | – | Theming | Examples |
Colors | Examples | – | Tables | Examples |
Headers | Examples | – | Styles | Examples |
Panels | Examples | – | Area | Examples |
Paragraphs | Examples | – | ||
Prefixes | Examples | – | ||
Progress Bars | Examples | – |
BubbleTea
bubbletea: The fun, functional and stateful way to build terminal apps. A Go framework based on The Elm Architecture. Bubble Tea is well-suited for simple and complex terminal applications, either inline, full-window, or a mix of both.
uitable
uitable: is a go library for representing data as tables for terminal applications. It provides primitives for sizing and wrapping columns to improve readability.
table = uitable.New()
table.MaxColWidth = 80
table.Wrap = true // wrap columns
for _, hacker := range hackers {
table.AddRow("Name:", hacker.Name)
table.AddRow("Birthday:", hacker.Birthday)
table.AddRow("Bio:", hacker.Bio)
table.AddRow("") // blank
}
fmt.Println(table)
Name: Ada Lovelace
Birthday: December 10, 1815
Bio: Ada was a British mathematician and writer, chiefly known for her work on
Charles Babbage's early mechanical general-purpose computer, the Analytical
Engine
Name: Alan Turing
Birthday: June 23, 1912
Bio: Alan was a British pioneering computer scientist, mathematician, logician,
cryptanalyst and theoretical biologist
tabular
Tabular simplifies printing ASCII tables from command line utilities without the need to pass large sets of data to it’s API.
package main
import (
"fmt"
"log"
"github.com/InVisionApp/tabular"
)
var tab tabular.Table
func init() {
tab = tabular.New()
tab.Col("env", "Environment", 14)
tab.Col("cls", "Cluster", 10)
tab.Col("svc", "Service", 15)
tab.Col("hst", "Database Host", 20)
tab.ColRJ("pct", "%CPU", 7)
}
var data = []struct {
e, c, s, d string
v float64
}{
{
e: "production",
c: "cluster-1",
s: "service-a",
d: "database-host-1",
v: 70.01,
},
{
e: "production",
c: "cluster-1",
s: "service-b",
d: "database-host-2",
v: 99.51,
},
{
e: "production",
c: "cluster-2",
s: "service-a",
d: "database-host-1",
v: 70.01,
},
{
e: "production",
c: "cluster-2",
s: "service-b",
d: "database-host-2",
v: 99.51,
},
}
func main() {
// Print a subset of columns (Environments and Clusters)
format := tab.Print("env", "cls")
for _, x := range data {
fmt.Printf(format, x.e, x.c)
}
// Print All Columns
format = tab.Print("*")
for _, x := range data {
fmt.Printf(format, x.e, x.c, x.s, x.d, x.v)
}
// Print All Columns to a custom destination such as a log
table := tab.Parse("*")
log.Println(table.Header)
log.Println(table.SubHeader)
for _, x := range data {
log.Printf(table.Format, x.e, x.c, x.s, x.d, x.v)
}
}
Environment Cluster
-------------- ----------
production cluster-1
production cluster-1
production cluster-2
production cluster-2
Environment Cluster Service Database Host %CPU
-------------- ---------- --------------- -------------------- -------
production cluster-1 service-a database-host-1 70.01
production cluster-1 service-b database-host-2 99.51
production cluster-2 service-a database-host-1 70.01
production cluster-2 service-b database-host-2 99.51
2018/05/14 11:19:41 Environment Cluster Service Database Host %CPU
2018/05/14 11:19:41 -------------- ---------- --------------- -------------------- -------
2018/05/14 11:19:41 production cluster-1 service-a database-host-1 70.01
2018/05/14 11:19:41 production cluster-1 service-b database-host-2 99.51
2018/05/14 11:19:41 production cluster-2 service-a database-host-1 70.01
2018/05/14 11:19:41 production cluster-2 service-b database-host-2 99.51
tabby
tabby: A tiny library for super simple Golang tables.
t := tabby.New()
t.AddHeader("NAME", "TITLE", "DEPARTMENT")
t.AddLine("John Smith", "Developer", "Engineering")
t.Print()
NAME TITLE DEPARTMENT
---- ----- ----------
John Smith Developer Engineering
Table
table: Print out tabular data on the command line using the ansi color esacape codes. Support for writing the ouput based on the fields in a struct and for defining and creating the table manully using the underlying object.
tab := table.Table{
Headers: []string{"something", "another"},
Rows: [][]string{
{"1", "2"},
{"3", "4"},
{"3", "a longer piece of text that should stretch"},
{"but this one is longer", "shorter now"},
},
}
err := tab.WriteTable(w, nil) // w is any io.Writer
