package main
import "fmt"
// basic type of some function to change behavior
type functype func(int, int) int
// my specific function which i want to change behavior via decorator
func sum(a, b int) int {
return a + b
}
// my decorator:
// basic sum function create new function with same type as the source
// but with new behavior: adds log message
func dec(prefix string, f functype) functype {
return func(x, y int) int {
res := f(x, y)
fmt.Printf("%s: %d\n", prefix, res)
return res
}
}
func main() {
// basic ussage
r1 := sum(10, 20)
fmt.Println(r1)
// create new sum function by decorator
sumWithLog := dec("suma", sum)
// same ussage as origin, but new behavior
r2 := sumWithLog(20, 30)
fmt.Println(r2)
}
go
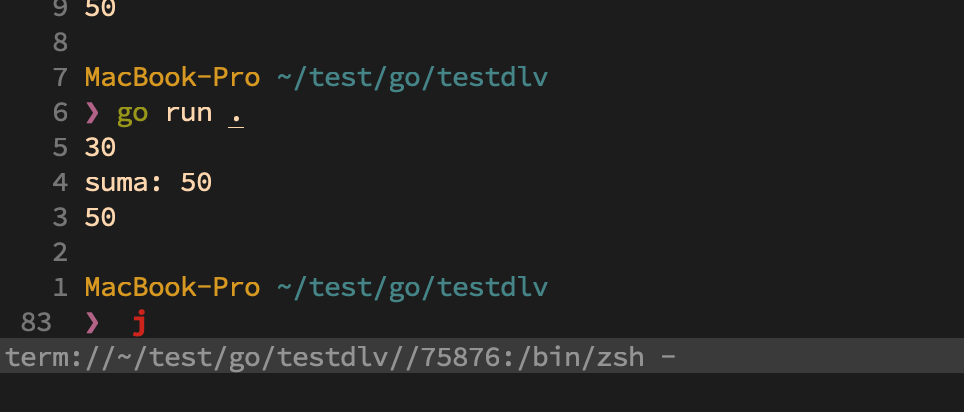