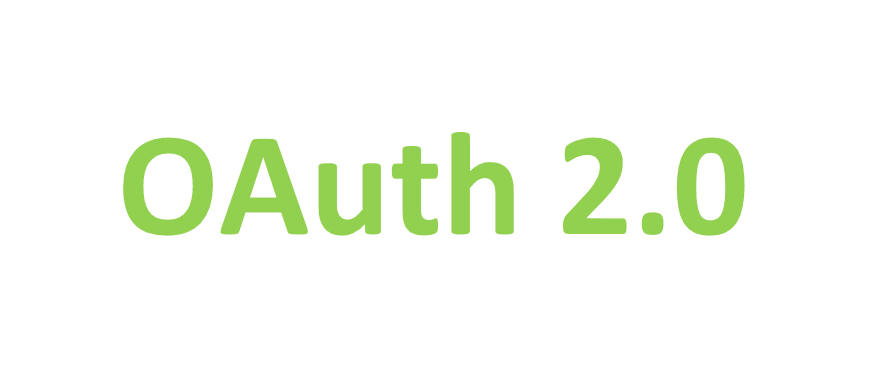
Fiber is an Express inspired web framework built on top of Fasthttp, the fastest HTTP engine for Go. Designed to ease things up for fast development with zero memory allocation and performance in mind.
Package goth provides a simple, clean, and idiomatic way to write authentication packages for Go web applications.
Unlike other similar packages, Goth, lets you write OAuth, OAuth2, or any other protocol providers, as long as they implement the Provider and Session interfaces.
All together in simple example
package main
import (
"log"
"github.com/gofiber/fiber/v2"
"github.com/markbates/goth"
"github.com/markbates/goth/providers/google"
gf "github.com/shareed2k/goth_fiber/v2"
)
const (
key = "googlekey"
sec = "googlesec"
)
func main() {
goth.UseProviders(
google.New(key, sec, "http://localhost:3000/auth/google/callback"),
)
app := fiber.New()
app.Get("/auth/:provider/callback", func(ctx *fiber.Ctx) error {
user, err := gf.CompleteUserAuth(ctx)
if err != nil {
return err
}
ctx.JSON(user)
return nil
})
app.Get("/logout/:provider", func(ctx *fiber.Ctx) error {
gf.Logout(ctx)
ctx.Redirect("/")
return nil
})
app.Get("/auth/:provider", func(ctx *fiber.Ctx) error {
if gothUser, err := gf.CompleteUserAuth(ctx); err == nil {
ctx.JSON(gothUser)
} else {
gf.BeginAuthHandler(ctx)
}
return nil
})
app.Get("/", func(ctx *fiber.Ctx) error {
ctx.Format("<p><a href='/auth/google'>google</a></p>")
return nil
})
log.Fatal(app.Listen(":3000"))
}