Spot is a simple, cross-platform, reactive GUI toolkit for Go using native widgets where available. It is designed to be easy to use and to provide a consistent API across different platforms.
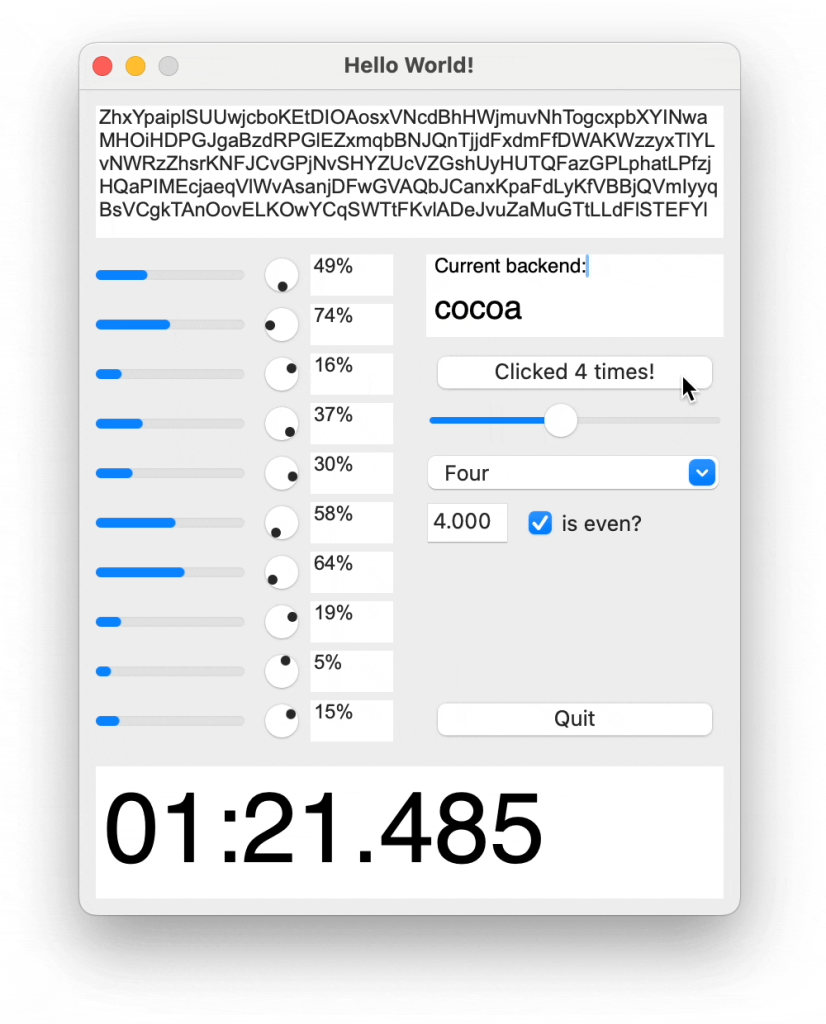
Features
- Simple: You can add Spot as a simple dependency to your project and start building your UI right away. No need to use additional tools or code generation steps. Just write Go code and get a native GUI application as a self-contained binary.
- Cross-platform: Spot uses native widgets where available and automatically selects the best backend for the platform you are running on at compile time. Currently, two backend implementations are provided: one based on FLTK using go-fltk and one based on Cocoa using (a modified version of) gocoa.
- Reactive: Spot automatically updates the UI when the state of the application changes. You just provide side-effect free rendering functions and manage the state of your application using the
UseState
hook. - Broad widget support: Spot provides a wide range of UI controls out of the box, including buttons, labels, text inputs, sliders, dropdowns, and more. See the full list: List of supported UI controls.
package main
import (
"fmt"
"github.com/roblillack/spot"
"github.com/roblillack/spot/ui"
)
func main() {
ui.Init()
spot.MountFn(func(ctx *spot.RenderContext) spot.Component {
counter, setCounter := spot.UseState[int](ctx, 0)
buttonTitle := "Click me!"
if counter > 0 {
buttonTitle = fmt.Sprintf("Clicked %d times!", counter)
}
return &ui.Window{
Title: "Hello World!",
Width: 200,
Height: 125,
Children: []spot.Component{
&ui.Button{
X: 25, Y: 50, Width: 150, Height: 25,
Title: buttonTitle,
OnClick: func() {
setCounter(counter + 1)
},
},
},
}
})
ui.Run()
}